WordPress Plugin Development Course for Designers – Part 2
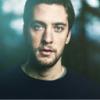
In the first part of our WordPress Plugin Development Course for Designers we learned about the importance of WordPress plugins for designers and its base structure. Today we are going to talk about the core WordPress theories and techniques required for creating design focused plugins.
Our final goal is to learn WordPress plugin development while integrating a jQuery slider into WordPress from scratch. Throughout this tutorial, we will be learning the necessary coding techniques using sample slider as the example. By the end of this tutorial, you will have a simple template which is common to most of the design based plugins.
Voting for the slider is still going on and SlidesJS is heading the list. So we will be using SlidesJS the slider for explaining necessary codes in this part.
So let’s get started.
What type of files are needed for Design Based Plugins?
Consider the following image which illustrates the files in a common plugin for creating design based components.
As you can see JavaScript and CSS will be kept in separate folders inside the main plugin. The main PHP file contains the plugin definition and the important initialization functions of the plugin. If you are developing a single file plugin, all the codes will be kept inside the main PHP file.
Although HTML is the most important type of file for you as designers, it doesn’t show up as separate files in the given screen. Actually there won’t be any HTML files inside the WordPress plugin. All the necessary HTML codes are generated through PHP files.
The preceding screen shows the basic folder and file structure of a WordPress plugin. We can add necessary libraries and additional files to modularize our plugin to increase the reusability.
Understanding the Components of Slider
Throughout this series we will be integrating a jQuery slider plugin to learn WordPress plugin development. Therefore it’s important to get used to the main components of a jQuery slider as shown in the following.
- jQuery library - We need a core library to develop plugins. jQuery is chosen as the core library for the plugin used in this series. You have the freedom to work with other core libraries such as Prototype, Dojo, MooTools, etc.
- jQuery plugin file - Every plugin has a main file which is built on top of the jQuery library. Usually we have all the codes for a jQuery plugin inside single JavaScript file.
- Intialization code - All the plugins need to be initialized at some point to make it work. Generally we use inline scripts in the HEAD section of the HTML document to include the initialization code.
- CSS files used for plugin - Most of the content or image sliders uses CSS to provide slide transitions and animations. Therefore we have CSS files specific to plugins to provide the default look and feel of the sliders.
- HTML or Images used for sliding - Final component of a slider is the actual text, image or HTML content which gets converted into a slider by the plugin. Unordered list with HTML and images is the most popular technique of defining the data required for the slider.
Now we have identified the main five components of a jQuery slider plugin. Let’s focus on learning how each of these components fit into an actual WordPress plugin.
Including Scripts in WordPress Plugins
Our slider plugin contains two JavaScript files at the moment and will have the third one for plugin initialization code. Most of the beginner developers makes the mistake of including scripts incorrectly inside WordPress plugins. So this section will focus on identifying the mistakes and learning how to avoid those mistakes in order to comply with the best practices.
I assume that most of the readers of this tutorial will be designers. So let’s see how we include script files in static HTML websites.
We basically hard code all the script files into HEAD section of the HTML document or footer section. This is not a problem at this stage since we are building static websites. Now let’s see how these scripts are included in WordPress plugin by developers who are not experienced in WordPress.
The Wrong Way of Including Scripts
Most inexperienced developers directly includes the scripts inside the PHP files using the echo statement as shown in the following code. This is considered as a bad practice in WordPress plugin development.
function fwds_scripts(){
echo "
";
echo "
";
}
The code prints the script files anywhere in the HTML file. It can be header, footer or body depending on location you place it. Even though we have a separate header for WordPress themes, it’s not wise to hard code the files in the header.
In static websites you can manage these files since you will be the only designer. WordPress has a plugin based architecture and hence anyone can write plugins. Therefore there is a high possibility of duplicating the same files with the technique mentioned above.
The Right Way of Including Scripts
Now we are going to look at the right way of including scripts, considering the limitations in above technique. WordPress came up with a concept called action hooks which will be executed at specific points of a request or when a specific event occurs in your system. You can define a functions to be called in these execution points.
It will take time to understand the complete concept of WordPress action hooks, even for an experienced WordPress developer. For the moment think of it as a code that calls PHP function at specific points. Consider the following code.
function my_custom_function() {
// Place the code to be executed here
}
add_action('action name', 'my_custom_function');
?>
We can replace the action name with predefined WordPress hooks or using our own custom hooks. You have to use different functions to different actions. In depth knowledge of action hooks will be provided in future tutorials when you get comfortable with basics of plugin development.
Now let’s switch back to the correct method for including scripts. WordPress provides a predefined hook called wp_enqueue_scripts
to include script files into pages. Inside our custom function we have to include the scripts one by one. Consider the following code.
add_action('wp_enqueue_scripts', 'fwds_scripts');
function fwds_scripts() {
wp_enqueue_script('jquery');
wp_register_script('slidesjs_core', plugins_url('js/jquery.slides.min.js', __FILE__),array("jquery"));
wp_enqueue_script('slidesjs_core');
wp_register_script('slidesjs_init', plugins_url('js/slidesjs.initialize.js', __FILE__));
wp_enqueue_script('slidesjs_init');
}
?>
Preceding code calls the fwds_scripts
function in the execution process using the wp_enqueue_scripts
action. I have used fwds(first web designer slider) as the prefix for my functions.
It is recommended to prefix your plugin functions with string related to your plugin name to avoid conflicts with functions in other plugins.
Inside fwds_scripts
, we have used two other functions for including script files called wp_enqueue_script
and wp_register_script
. wp_enqueue_script
is used to include the script file to the HTML document. jQuery is one of the libraries which comes in-built with WordPress and hence we can include it directly as shown in the code. Latest version of jQuery will be included in this technique.
wp_register_script
is used to register a script file into WordPress. We have to register the slider plugin file and initialization file since it is not built-in with WordPress. First parameter is a unique name to register your library. Next you have to provide the path of the js file. plugins_url
function will provide the URL of the plugin folder.
Third parameter specifies the dependent libraries. In this scenario, our plugin file depends on the jQuery library. We don’t have to manage dependencies with this technique. Before registering any script, WordPress checks whether it has been already registered using the given unique name. File is loaded only when it’s not loaded previously. So it will prevent the file duplication discussed in the earlier section.
If you register the same script file with different names, WordPress will not be able to handle the file duplication.
SlidesJs core plugin file is named as jquery.slides.min.js
and we have included it in the preceding code. Also we have included the initialization part of the library inside another js file called slidesjs.initialize.js
. Following code contains the initialization code inside the js file.
jQuery(function() {
jQuery('#slides').slidesjs({
width: 940,
height: 528,
navigation: false
});
});
There are various other recommended ways of including scripts files without using wp_enqueue_scripts
action hook. Technique discussed here is good enough for us to learn plugin development as designers. I will also cover the other techniques when required.
Including Styles in WordPress Plugins
Usually most of the jQuery plugins comes up with built-in CSS file to provide its default look and feel. As designers we are used to including CSS files directly in the HTML document. So the beginners of WordPress plugin development tries to apply the same method as I discussed in JavaScript section.
The Wrong Way of Including Styles
Consider the following code to see how beginners include CSS files in WordPress plugins.
function fwds_styles(){
echo
";
echo
";
}
The code will include style files everywhere in the HTML document without considering file duplication. This works exactly the same way how JavaScript worked.
The Right Way of Including Styles
Recommended method of including style files is exactly similar to the method we discussed for JavaScript files. wp_enqueue_scripts
action will be used again with different function name to include the styles as shown in the following code.
add_action('wp_enqueue_scripts', 'fwds_styles');
function fwds_styles() {
wp_register_style('slidesjs_example', plugins_url('css/example.css', __FILE__));
wp_enqueue_style('slidesjs_example');
wp_register_style('slidesjs_fonts', plugins_url('css/font-awesome.min.css', __FILE__));
wp_enqueue_style('slidesjs_fonts');
}
We have to use wp_register_style
and wp_enqueue_style
in this case instead of script functions. Usage and parameters are exactly similar to script functions.
In this part, we are using SlidesJS for implementing the slider plugin. SlidesJS comes up with two CSS files for styles neccessary for look and feel of the presentation and including fonts.
Now we know the basics of including scripts and styles of the jQuery plugin inside the WordPress plugin. Generally we place the initialization code for a plugin, inline within script tags in static HTML files. With WordPress, it’s better to add it to an existing js file or include in a new js file and load using the recommended way.
Assigning Content to Slider Using Shortcodes
Shortcodes can be considered as an alias for reusable piece of code. First we have to define the common code inside a PHP function. Then we can access it anywhere in themes, plugins or page editor by using the alias given for function. Consider the code below for most basic use of WordPress shortcode.
add_shortcode("my_shortcode", "my_shortcode_function");
function my_shortcode_function() {
return "
Hello Shortcodes
";
}
WordPress provides a built-in method called add_shortcode
for defining shortcodes. First parameter is the name (alias) of the shortcode. Second parameter contains the function to be executed when the shortcode is requested. We return a simple string from the shortcode.
Some developers like to directly print the output of the shortcode inside the function. You should always prefer returning output from function and printing externally as shown in the preceding example.
Then you can use [my_shortcode/] to refer the common code in multiple locations. Folowing screen shows how you can use a shortcode inside the post or page editor in WordPress.
Shortcodes can be used inside theme or plugin files with do_shortcode function as shown below.
Advanced Usage of Shortcodes
In the previous example, we used the most basic form of shortcode. We can also have attributes and content in shortcodes to add additional features. Consider the following shortcode with attributes and content.
[my_advanced_shortcode name="Nimesh" age="27"] Description about me [/my_advanced_shortcode]
These types of shortcodes contain opening and closing shortcode tags. Information placed between the opening and closing tags is considered content and key-value pairs inside the opening tag is considered as attributes. Following code shows how we can extract these information inside the actual shortcode function.
function my_advanced_shortcode_handler( $atts, $content = null ) {
extract( shortcode_atts( array(
'name' => 'Rakhitha',
'age' => '20'
), $atts ) );
echo $name;
echo $age;
echo $content;
}
Content and attributes of the shortcodes will passed as the default parameters to shortcode function. We can directly use the content using the $content
variable. Here you will get “Description about me” as the content.
Handling attributes is not straightforward as content. We have to extract each attribute from the $attr
array. We can use the extract function as shown here. We define all the attribute with the default values. If attributes are supplied with shortcodes, default value will be replaced by passes value. Finally you can access these attributes using $name
and $age
.
Integrating Slider Using Shortcodes
In the previous sections we discussed how scripts, styles and initialization code fits into a WordPress plugin. Final part of the slider will be the actual HTML or images used as the content for sliding. Shortcodes will be the ideal method for providing slider content. Now let’s see how images fit into the shortcode.
add_shortcode("1wd_slider", "fwds_display_slider");
function fwds_display_slider() {
$plugins_url = plugins_url();
echo '
';
}
Now we have integrated all the necessary parts into WordPress plugin. But we still have a slider with static content. Real power of plugin comes when we can add the slider content dynamically and create multiple sliders to use in multiple locations.
Now you can download the static version of WordPress slider plugin here. Copy the downloaded plugin into plugins folder and activate using the admin dashboard. Then create a post or page and insert [1wd_slider/]
in the TinyMCE editor and click the publish button to see the slider in action. You will be able to use the navigation button to see how sliding works.
So try out the static version of slider plugin for multiple jQuery libraries using the following template we created throughout this tutorial.
/*
Plugin Name: 1WD Slider
Plugin URI: http://1stwebdesigner.com/
Description: Slider Component for WordPress
Version: 1.0
Author: Rakhitha Nimesh
Author URI: http://1stwebdesigner.com/
License: GPLv2 or later
*/
function fwds_slider_activation() {
}
register_activation_hook(__FILE__, 'fwds_slider_activation');
function fwds_slider_deactivation() {
}
register_deactivation_hook(__FILE__, 'fwds_slider_deactivation');
add_action('wp_enqueue_scripts', 'fwds_scripts');
function fwds_scripts() {
wp_enqueue_script('jquery');
wp_register_script('slidesjs_core', plugins_url('js/jquery.slides.min.js', __FILE__),array("jquery"));
wp_enqueue_script('slidesjs_core');
wp_register_script('slidesjs_init', plugins_url('js/slidesjs.initialize.js', __FILE__));
wp_enqueue_script('slidesjs_init');
}
add_action('wp_enqueue_scripts', 'fwds_styles');
function fwds_styles() {
wp_register_style('slidesjs_example', plugins_url('css/example.css', __FILE__));
wp_enqueue_style('slidesjs_example');
wp_register_style('slidesjs_fonts', plugins_url('css/font-awesome.min.css', __FILE__));
wp_enqueue_style('slidesjs_fonts');
}
add_shortcode("1wd_slider", "fwds_display_slider");
function fwds_display_slider() {
$plugins_url = plugins_url();
echo '
';
}
?>
Demo and Challenge
If you want to see the working version of what we have created so far, you can check the demo here.
Now, here’s a challenge to you:
If you have installed the plugin and checked, you will notice that it occupies a full width of 940px. And on our demo site the slider has overlapped the sidebar because of this. Question is, can you make it responsive on your own? It’s easy!
The answer will be revealed on the next instalment of our WordPress Plugin Development Course for Designers, watch out for it!
Up Next
In the next part we will be creating the actual integration of jQuery plugin into WordPress. Instead of just hard coding HTML or images inside unordered lists, we will be creating sections to upload images dynamically and create multiple sliders by selecting preferred images.
Until then I would like to suggest following resources to get an idea about the contents in the next part of this tutorial series.
Now it’s your turn.
Integrate various other sliders using the technique we discussed today. Now don’t worry about sliders being static at the moment. I will explain how to make it dynamic and reusable in the next part.
Let me know about the type of sliders you tried and any difficulties you faced in applying the discussed technique.
- Login om te reageren