Creating Your Own CMS Part 1 – Secure Login
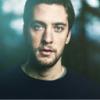
Have you ever built a site to realize it is a pain to manage? There are a lot of CMS options out there but some of them cost money, some are hard to learn, some your hosting company might not support, and well sometimes they just don’t have everything thing that you want. So today I am going to start to teach you how to create your own simple CMS. Today I will get into how you can find a design and get your secure login setup. This will be a series of blogs but to be honest I am not sure how advanced I will be going. If I wanted to spill it all and tell you how to build the CMS that my company uses then it might take a few blogs.
Design of Your Own CMS
This may or may not be important to you. But when it comes down to it you will most likely be much more proud of your CMS with a nice design and design is where the usability starts. I could programming some amazing things but without a design I am limiting myself and wasting a lot of time. I have created a few CMS’s from scratch but I found out that when you give me a design for a CMS it not only comes out a lot neater but I can produce it much faster.
Here is the one that I chose as well as a few others to browse through.
I am creating this CMS as I type this so you guys are looking at it first hand. That is also the main reason I am not sure how many advanced parts we will have but I am willing to take requests while I work.
A Few Things To Have Before You Begin the Login
If you haven’t already setup the database then you can refer to one of my recent posts “Getting Started With MySQL DB and PHP with PHPMyAdmin”. Along with the database it is nice to have a database class file and a database connection file. These are two files that you will be using on each project you do so it is nice to have them on hand. The db.php can just go in the htdocs/ and the db.class.php should go in the htdocs/classes/ folder.
People do their file structure differently but for me I have an Ubuntu server with each site in the home folder like: /home/www.llafl.com/htdocs/
Within the htdocs/ we will want a few different folders as well.
- admin/
- classes/
- css/
- images/
- includes/
- lib/
- markitup/
- cache/
- classes/
- css/
- images/
- includes/
- lib/
Most of this layout is pretty self-explanatory. You would have your index and other content files in the htdocs/ and in the htdocs/admin/ would be all of you admin content. Then the rest of the folders are all labeled for exactly what should be going in them. The cache/ folder can be used for several different items depending on the type of website that we are building.
Obviously this is not required nor any sort of standard this is just how I like to layout my sites file system to try to keep things neat.
Here the folder “markitup” contains a jquery markup editor which I actually haven’t used until now. I usually use ckeditor for a full featured easy to use editor.
Secure Login Code
With a secure login we will want to use sessions. You can get into more advanced parts with heavier use in cookies also but for now I am just going to show you a very basic secure login with a timeout on it. First though we need to make sure that when people try to go anywhere on our site that they will get sent to the login if they are not already logged in. Se we should put this in the header.php because that is included on every page in the admin, and we can do it like this.
if(!$_SESSION['llafl_admin'])
{
$_SESSION['REQUEST_URI'] = $_SERVER['REQUEST_URI']; //store the page they were trying to access
header('Location: /admin/login.php');
exit;
}
?>
You can see here I am checking for a session variable which I have named ‘llafl_admin’. It is best to have a distinct session because if not then you run the chance of some other site having the same session variable. Like if we just made the variable ‘admin’, it is so generic it could be anywhere. So since we have a check like this that means that when someone is logged in then they should have a session variable ‘llafl_admin’, which we will do like this.
if($_SESSION['failed']=='yes')
echo '
'; // display failed login if need be
?>
That is a a basic login form and below is the code to handle when it is submitted.
if($_POST)
{
if(!$_POST['username'] || !$_POST['password'])
$_SESSION['failed']='yes';
else
{
$username = stripslashes($_POST['username']);
$password = md5($_POST['password']);
$u_q = $db->query("SELECT * FROM users WHERE username='$username' AND password='$password' LIMIT 1");
$u = $db->fetch_assoc($u_q);
if($u['id'])
{
$_SESSION['llafl_admin'] = $u['access_level'];
$_SESSION['started'] = time();
foreach($u as $key => $value)
$_SESSION[$key] = stripslashes($value);
$last_login = date('c',time());
$db->query("UPDATE users SET last_login='$last_login' WHERE id='".$_SESSION['id']."' LIMIT 1"); // on successful login update users last login
header('Location: '.$_SESSION['REQUEST_URI']); //if they successfully log in then send them to the url they requested which is something I have set in the header
exit;
}
else
$_SESSION['failed']='yes'; // if they failed to login this will set a session var that will trigger an error message
}
}
?>
You can see here this script checks to make sure that both inputs were indeed submitted and if they were then it goes on to check to see if they are in fact part of a legit login. Then if it is successful then the appropriate session variable are set. (You notice we do a stripslashes to sanitize the username and md5 will sanitize the password and make sure that it is not stored human readable in case the database were to be compromised.) And if not then a session variable for ‘failed’ will be set and when the login form reloads it will trigger an error message. There are many more security levels that you can add onto this but this is a very basic and secure login that will work for our purposes.
If you are confused by any of the database aspects then refer back to this post.
- Login om te reageren